Classification of patterns in software development
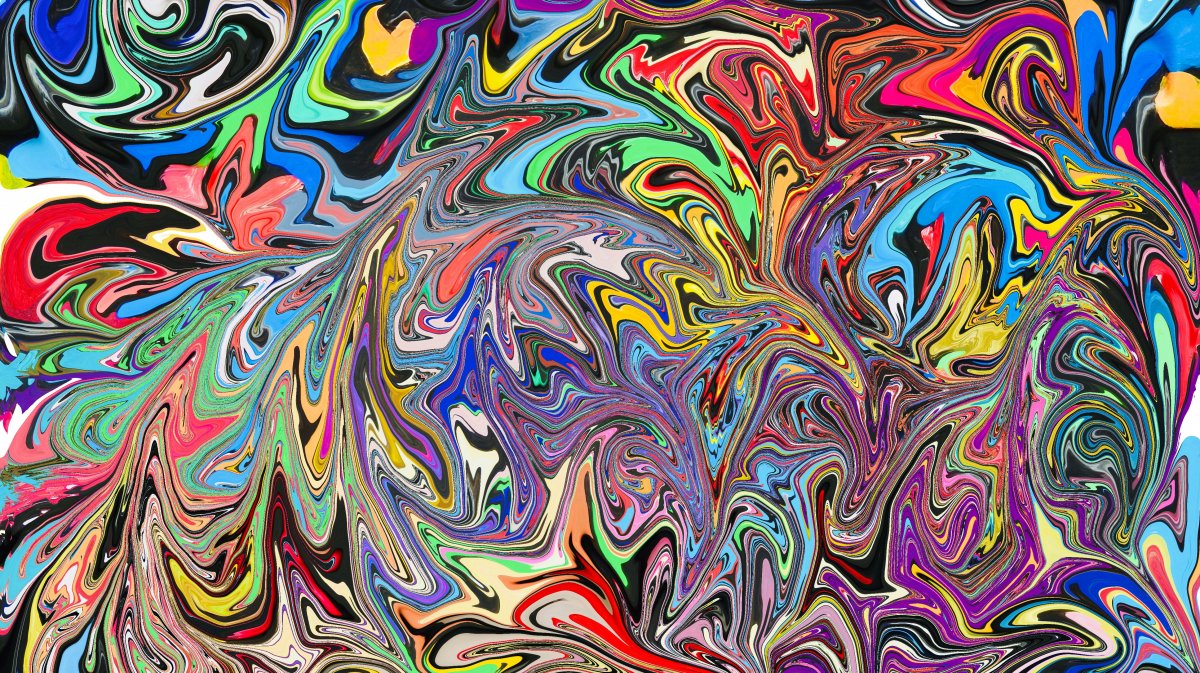
Patterns can be classified just like design patterns, and the book Pattern-Oriented Software Architecture provides a good starting point.
In my last article I presented the classification of design patterns based on the seminal book Design Patterns: Elements of Reusable Object-Oriented Software. Today I present a more general classification of patterns based on the second seminal book, Pattern-Oriented Software Architecture, Volume 1.
The classification in my last article “Classification of Design Patterns in Software Development” referred to design patterns, but this article “Classification of Patterns” is about patterns. This is intentional, because the classification of “Pattern-Oriented Software Architecture, Volume 1” (POSA 1 for short) is more general than that of “Design Patterns: Elements of Reusable Object-Oriented Software”. To make it short, today’s classification includes the last one.
Pattern-Oriented Software Architecture, Volume 1
POSA 1 uses two types of classification. It classifies the patterns by their structural category and by their problem category. Before going into the two classifications, I would like to say a few words about the bold patterns in the table, all of which I will discuss.
Rainer Grimm has been working as a software architect, team leader and training manager for many years. He likes to write articles on the programming languages C++, Python and Haskell, but also likes to speak frequently at specialist conferences. On his blog Modernes C++ he deals intensively with his passion for C++.
The Proxy, Publish-Subscriber, and Counted Pointer design patterns are special. Proxy is already part of the book “Design Patterns: Elements of Reusable Object-Oriented Software” and Publish-Subscriber is very similar to the Observer pattern, which is also in the book mentioned earlier. You should also know and use the counter pointer idiom. In C++11 we call it std::shared_ptr
.
Structural Categories
The structural categorization is a categorization according to its scope and abstraction:
- architectural pattern describe the basic structure of the entire software system. They are often based on design patterns.
- design pattern define the interaction of the components and focus on subsystems.
- A idiom is an implementation of an architecture or design pattern in a concrete programming language. The popular idiom in C++ is Resource Acquisition Is Initialization (RAII). Containers, smart pointers and locks implement this.
I’d like to recap my thoughts on architectural patterns, design patterns, and idioms:
- The structural categories range from abstract to concrete. Idioms are the most concrete.
- They work on the macro level (architectural patterns), the micro level (design patterns) and the programming language (idioms).
- Architectural patterns focus on the system, design patterns on the subsystems, and idioms on the programming language.
Let’s look at the different problem categories.
problem categories
In “Pattern-Oriented Software Architecture, Volume 1” there are ten different problem categories. I will present them and their patterns in a compact form before delving deeper into some of them in the next few articles.
- From Mud to Structure offers a controlled decomposition of an overall system task into cooperating subsystems.
- layers: Break down a task into layers. Each layer has a specific responsibility and provides a service to a higher layer.
- Pipes and Filters: Decompose a task that performs complex processing into a series of separate elements that can be reused. This can improve performance, scalability, and reusability by allowing task elements that perform the processing to be deployed and scaled independently (
- blackboard: Several specialized subsystems combine their knowledge to create a possible partial solution. It is used for problems for which no deterministic solution is known.
- Distributed Systems builds systems whose components reside in different processes or address spaces.
- brokers: Structures distributed software systems that interact with remote service calls. He is responsible for the coordination of the communication, its results and exceptions.
- Interactive Systems builds a system with a human-computer interaction.
- Model View Controller (MVC): Divides the program logic of a user interface into the individual components model, view and controller. The model manages the data and rules of the application. The view renders the data and the controller interacts with the user.
- Presentation Abstraction Controller (PAC): is similar to MVC. In contrast to the MVC, the PAC has a hierarchical structure of agents, with each agent consisting of a presentation, abstraction and control part.
- Adaptable Systems makes an application extensible and adaptable to new requirements.
- microkernel: Separates a minimal feature core from advanced functionality.
- reflection: Splits a system into two parts: a meta level and a base level. The meta level supports the system properties and makes it self-reflective. The base level includes the application logic.
- Structural Decomposition breaks down a system into subsystems and complex components, into appropriately cooperating components.
- whole part: Builds an aggregation of components to make the parts a whole. Provides a common interface for the parts. This design pattern is the composite pattern from the book Design Patterns: Elements of Reusable Object-Oriented Software.
Organization of Work
works together with several components to offer a complex service.
- master slave: The master distributes its work to its slaves and collects the results from them.
- access control protects and controls access to services and components:
- proxy: It is a wrapper that is called by the client to access the actual object. A proxy usually adds additional logic like caching, security or encryption. This additional logic is hidden from the client.
- management manages a homogeneous set of objects, services and components in their entirety.
- Command Processor: Turns commands into objects so that their execution can be scheduled, saved, or later undone.
- view handler: …helps to manage all views that a software system provides. A view handler component allows clients to open, manipulate and dispose of views. It also coordinates dependencies between views and organizes their update. ([Pattern-OrientedSoftwareArchitectureVolume1)[Pattern-OrientedSoftwareArchitectureVolume1)
- communications Organizes the communication between the components.
- Forwarder receiver: Provides transparent interprocess communication for software systems with a peer-to-peer interaction model. It introduces forwarders and receivers to decouple peers from the underlying communication mechanisms. (Pattern-Oriented Software Architecture, Volume 1)
- Client dispatcher server: Introduces the dispatcher as a layer between clients and servers. The dispatcher ensures transparency between the clients and the servers.
- Publish Subscriber: Allows the publisher to automatically notify all subscribers. This design pattern is the Observer Design Pattern from the book “Design Patterns: Elements of Reusable Object-Oriented Software”.
resource management
helps manage shared components and objects.
- counted pointers: Introduces a reference count for dynamically allocated shared objects. std::shared_ptr is the prominent example in C++.
What’s next?
This article ends my introduction to patterns. In my next article I will present the structure of a design pattern based on the book “Design Patterns: Elements of Reusable Object-Oriented Software“.